Reminder: Readings are your responsibility. You will be expected to come to class prepared, having read the material, and ready to participate in the discussion
Logistics
- What is move_base?
- Using the map and the current estimated position (AMCL)
- Plan a route to a destination, avoiding obstacles
- Why is an estimate needed?
- Purely for AMCL
- Not related to navigation
- 2D Destination?
- Why not 3D, after all Gazebo is a 3D world
What do we mean by Complex Behavior?
- Obey a high level goal by creating subgoals, and responding to real time events
move_base
behaviors are pretty complex
- A lot can be learned from Computer Games and the behavior of “Non Player Characters”
- Obviously an open ended question with many different approaches
Discussion: Which teams have an example of requiring "complex behavior", or of apparent 'inteligence'. What were you planning to do?
Example scenario
- Lets say we want to implement the following “complex behavior”:
If Robot is stuck, then try the following, one after the other until the robot is unstuck: a) Conservative reset; b) clearing rotation; c) aggressive reset; d) clearing rotation. If after all of those the robot is still stuck, then abort the navigation
Discussion: How would you implement that?
Introduction to Finite State Machines
- Finite Sate Machines also known as FSA - Finite State Automata or Deterministic Finite State Automata
- Basic and very useful way to control behaviors
- And with lots of other applications! (See Regular Expressions and Cosi 130A)
- You have seen it before, whether you identified it as an FSM or not`
Essential features:
- Named nodes, corresponding to states
- Edges connecting nodes, corresponding to “inputs”
- Initial, Final and current states
Algorithm
- Start in the initial state
- Receive an input
- Follow the one edge out of that state, given that input
- Repeat until you get to final state
NoticeDepending on the scenario, the states, transitions and inputs can be very different.
Example of a real world FSM used in ROS
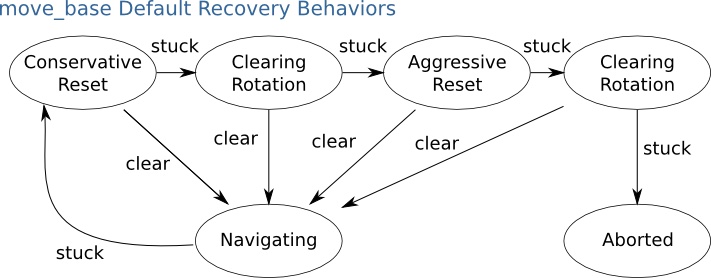
Implementations
- So simple that you don’t really need a package
- But one part of the diagram can’t be implemented as a basic FSM
- We can either modify it or use a more fancy kind of finite state machine
Discussion: Can you tell what the error is in the diagram (I didn't notice it before I actually went to implement it)
Using transitions
python package
- You can decide whether you want to use a package or not
- My favorite package is: pytransitions
- And an implementation of the above FSM with pytransitions in
samples
fsm_example.py
Discussion: What are the tradeoffs in using a package or not?
Move Base as a Finite State Machine
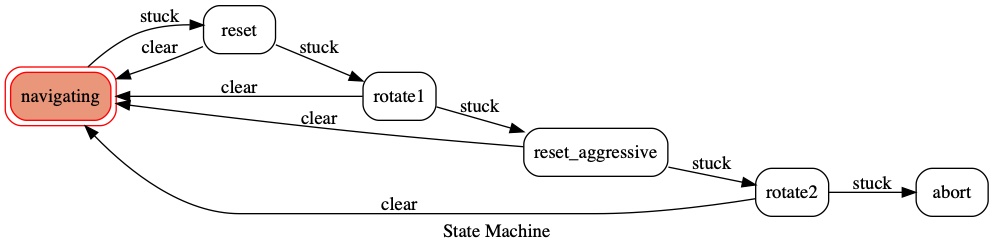
For further study
Joints and Links
What is URDF?
- Uniform Robot Definition Format
- Defines a collection of links held together by joints
- Joints
- Are not “visible” - all they do is to show how two links move relative to each other
- See urdf documentation for all the details
- Links
- Are “visible”
- Can have many other properties
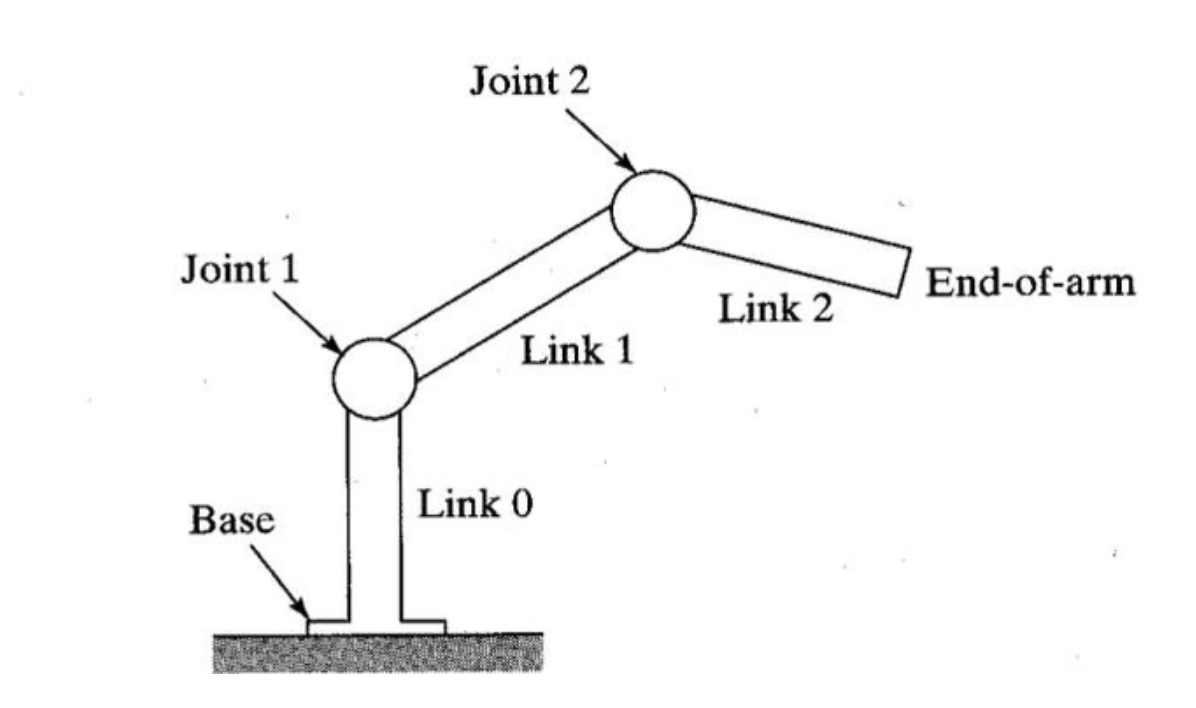
URDF Example
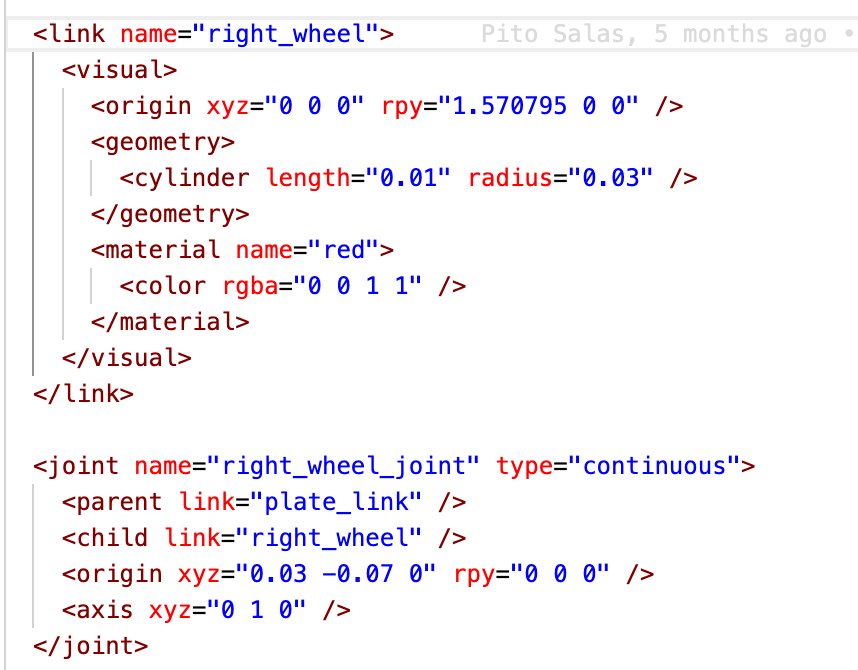
Joint Types
- revolute - a hinge joint that rotates along the axis and has a limited range specified by the upper and lower limits.
- continuous - a continuous hinge joint that rotates around the axis and has no upper and lower limits.
- prismatic - a sliding joint that slides along the axis, and has a limited range specified by the upper and lower limits.
- fixed - This is not really a joint because it cannot move. All degrees of freedom are locked. This type of joint does not require the axis, calibration, dynamics, limits or safety_controller.
- floating - This joint allows motion for all 6 degrees of freedom.
- planar - This joint allows motion in a plane perpendicular to the axis.
.launch file concerns
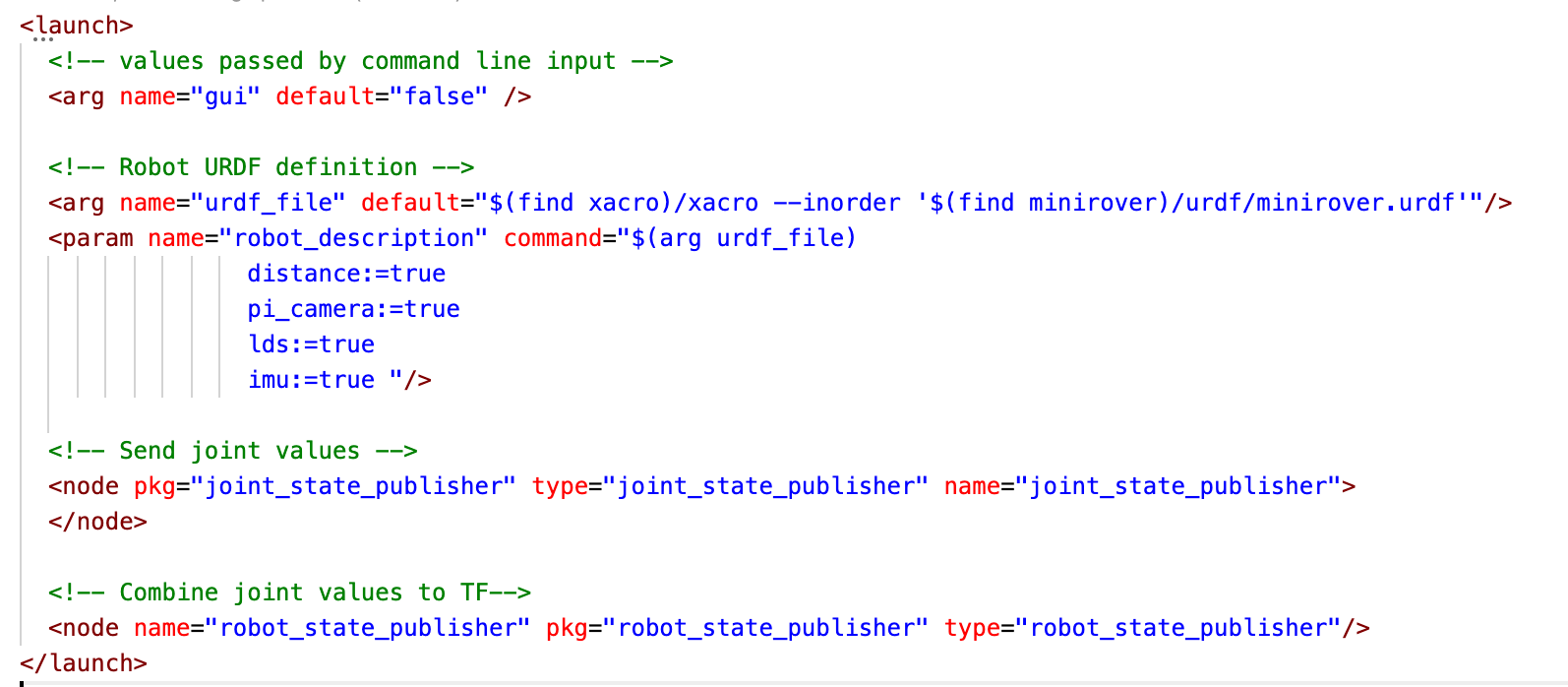
URDF Roles
- Describes geometry of a robot
- Names various links
- There are others
robot_state_publisher
- Looks at the urdf and publishes all the tfs which are statically tied to the base_link
- Using urdf, publishes
static_tf
joint_state_publisher
- Looks at dynamic links (those that can move) and updates the tf as they move
- What would cause them to move?
Thank you. Questions?
(random Image from picsum.photos)